- Curlis a tool to transfer data from or to a server, using one of the supported protocols (HTTP, HTTPS, FTP, FTPS, GOPHER, DICT, TELNET, LDAP or FILE).
- Curl is a tool to transfer data from or to a server, using one of the supported protocols (DICT, FILE, FTP, FTPS, GOPHER, HTTP, HTTPS, IMAP, IMAPS, LDAP, LDAPS, MQTT, POP3, POP3S, RTMP, RTMPS, RTSP, SCP, SFTP, SMB, SMBS, SMTP, SMTPS, TELNET and TFTP). The command is designed to work without user interaction.
Curl FAQ: How do I use curl to get the headers from a website URL? Short answer: Use curl's -I option, like this: $ curl -I URL Here's a specific example, including a real URL and results.
A reference guide to making GET, POST, PUT, PATCH, and DELETE API calls through the command line via cURL and their Postman equivalents.
Postman is an API testing environment. cURL is a command line tool for transfering data via URLs. When it comes to REST APIs, we can use Postman as a GUI (graphical user interface) and cURL as a CLI (command line interface) to do the same tasks.
Prerequisites
If you don't yet understand REST or know how to use REST APIs, please read Understanding REST and REST APIs.
Goals
I'm going to demonstrate how to do GET
, POST
, PUT
, PATCH
, and DELETE
requests via Postman and cURL. If you don't have Postman, simply download it from the website. cURL should already be installed in your macOS or Linux environment.
Endpoints
I'm going to use JSON Placeholder, an awesome example site for testing API calls. You can follow along and paste all the commands into your terminal to see what response you get.
Here is the map of methods to endpoints we'll be using. /posts
means all, and the 1
in /posts/1
represents /posts/{id}
, so ID number1
.
Method | Endpoint |
---|---|
GET | https://jsonplaceholder.typicode.com/posts |
POST | https://jsonplaceholder.typicode.com/posts |
PUT | https://jsonplaceholder.typicode.com/posts/1 |
PATCH | https://jsonplaceholder.typicode.com/posts/1 |
DELETE | https://jsonplaceholder.typicode.com/posts/1 |
You can click those URLs to see the GET values they provide to the browser. You can use the browser for GET, but you'll have to use cURL or Postman to POST, PUT, PATCH or DELETE. Download adobe lightroom mac free.
cURL CLI arguments
Here are a few cURL argument we'll pass with our requests. All requests will simply be curl
followed by the argument and data to pass.
-X --request
- Custom request method-d --data
- Sends the specified data-H --header
- Sends headers-i --include
- Display response headers
GET
GET retrieves data.
You can also use curl -i
to get more information from the headers.

All you have to do for Postman is paste the URL, select GET, and send.
POST
POST creates a new resource. It is non-idempotent, meaning that two identical POST requests will create two new resources.
There are two ways to do this via Postman. After selecting POST, you can go to Body, select x-www-form-urlencoded
, and type each individual value in. If you go to Headers, you'll see Content-Type: application/x-www-form-urlencoded
.
Or you can go to Body, select raw, select JSON
, and send the actual JSON you intend to send. If you go to Headers, you'll see Content-Type: application/json
.
PUT
PUT updates an existing resource. It is idempotent, meaning that two identical PUT requests will modify the same resource. A PUT request requires the entire body to be sent through; if any data is missing, that data will be wiped (except automatic values like auto-incrementing IDs and timestamps).
Sending the values is the same as with POST.
PATCH
PATCH updates an existing resource, and does not require sending the entire body with the request.
No change to sending the values.
DELETE

DELETE removes a resource.
No values to send.
Authentication
If you need to send additional headers, like Authorization: Bearer
or x-jwt-assertion
for JWT-based authentication, you can do it through cURL like this.
In Postman, you'll go to Headers and add Authorization
as the key and Bearer <JWT_TOKEN>
as the value to send authentication values. You can also go to Headers, click Presets, Manage Presets, and put your own reusable variables in for any headers or values you'll be reusing a lot.
Conclusion
This guide provides all the basics for getting started with testing your APIs, either through Postman's GUI or cURL's CLI, using JSON or urlencoded form data.
curl is a a command line tool that allows to transfer data across the network.
It supports lots of protocols out of the box, including HTTP, HTTPS, FTP, FTPS, SFTP, IMAP, SMTP, POP3, and many more.
When it comes to debugging network requests, curl is one of the best tools you can find.
It’s one of those tools that once you know how to use you always get back to. A programmer’s best friend.
It’s universal, it runs on Linux, Mac, Windows. Refer to the official installation guide to install it on your system.
Fun fact: the author and maintainer of curl, swedish, was awarded by the king of Sweden for the contributions that his work (curl and libcurl) did to the computing world.
Let’s dive into some of the commands and operations that you are most likely to want to perform when working with HTTP requests.
Those examples involve working with HTTP, the most popular protocol.
Perform an HTTP GET request
When you perform a request, curl will return the body of the response:
Get the HTTP response headers
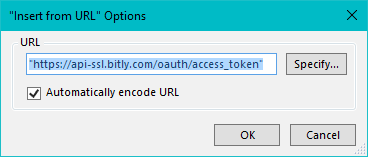
By default the response headers are hidden in the output of curl. To show them, use the i
option:
Only get the HTTP response headers
Using the I
option, you can get only the headers, and not the response body:
Perform an HTTP POST request
The X
option lets you change the HTTP method used. By default, GET is used, and it’s the same as writing
Using -X POST
will perform a POST request.
You can perform a POST request passing data URL encoded:
In this case, the application/x-www-form-urlencoded
Content-Type is sent.
Perform an HTTP POST request sending JSON
Instead of posting data URL-encoded, like in the example above, you might want to send JSON.
In this case you need to explicitly set the Content-Type header, by using the H
option:
You can also send a JSON file from your disk:
Perform an HTTP PUT request
Cd spin doctor mac free download. The concept is the same as for POST requests, just change the HTTP method using -X PUT
Follow a redirect
A redirect response like 301, which specifies the Location
response header, can be automatically followed by specifying the L
option:
will not follow automatically to the HTTPS version which I set up to redirect to, but this will:
Store the response to a file
Using the o
option you can tell curl to save the response to a file:
You can also just save a file by its name on the server, using the O
option:
Curl Url
Using HTTP authentication
If a resource requires Basic HTTP Authentication, you can use the u
option to pass the user:password values:
Set a different User Agent
The user agent tells the server which client is performing the request. By default curl sends the curl/<version>
user agent, like: curl/7.54.0
.
You can specify a different user agent using the --user-agent
option:
Curl Url Post
Inspecting all the details of the request and the response
Use the --verbose
option to make curl output all the details of the request, and the response:
Copying any browser network request to a curl command
When inspecting any network request using the Chrome Developer Tools, you have the option to copy that request to a curl request:
